How to create custom radio buttons in HTML using CSS and jQuery
Topic: JavaScript / jQueryPrev|Next
Answer: Use the CSS :checked
Pseudo-class with jQuery
If try to style radio buttons directly using CSS properties like background
or border
it will not work, because most of the form elements are native part of the browsers and does not accept so much visual styling. So we have to find another way of styling radio buttons.
In this tutorial we will create custom radio buttons with the help of CSS and little bit of jQuery. There are many other solutions available like jQuery plug-ins to customize radio buttons but they are unnecessarily complex and not so easy to implement. Here we will simulate the effect of custom radio buttons with the minimum effort that works like a charm and very easy to implement in any project. It is highly compatible across browsers, even works perfectly in Internet Explorer 7.
The HTML Code
Create radio button like you do in regular HTML and place it any where, but do not forget to put the name
attribute, because this solution depends on the name
attributes of the radio buttons, which is also required if you want to put your radio buttons in real action.
Example
Try this code »<form action="action.php" method="post">
<h3>What Is Your Favorite Web Browser</h3>
<label><input type="radio" name="browser" checked="checked" value="firefox"> Firefox</label>
<label><input type="radio" name="browser" value="chrome"> Chrome</label>
<label><input type="radio" name="browser" value="safari"> Safari</label>
</form>
Custom Radio Button Example Images
For the sake of simplicity, we have used separate images to specify the different states of a radio button like normal, hover, selected etc. but it is recommended to combine all these images in an image sprite form before using this solution in a real project for better performance and minimizing the HTTP request. However if you download the source code of this custom radio buttons example it will contain both the simple and sprite version. Let's take a look at the following images.
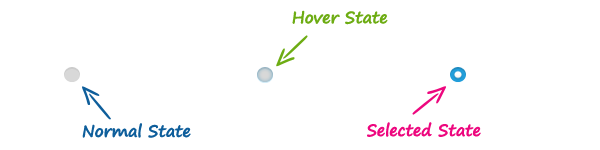
The CSS Code
What we are trying to do with the CSS is hide the default radio buttons with the help of CSS opacity
property and show our custom radio buttons in place of them.
Now place the following style rules inside the head section of your HTML document.
Example
Try this code »<style>
.custom-radio{
width: 16px;
height: 16px;
display: inline-block;
position: relative;
z-index: 1;
top: 3px;
background: url("radio.png") no-repeat;
}
.custom-radio:hover{
background: url("radio-hover.png") no-repeat;
}
.custom-radio.selected{
background: url("radio-selected.png") no-repeat;
}
.custom-radio input[type="radio"]{
margin: 1px;
position: absolute;
z-index: 2;
cursor: pointer;
outline: none;
opacity: 0;
}
</style>
You might be thinking we haven't used these classes anywhere in the HTML code; actually these classes will be added to the DOM by the jQuery at run time.
The jQuery Code
Our customRadio()
function simply wrap the default radio buttons in a <span>
element and apply the class .custom-radio
on it. Once the .custom-radio
class is applied on the <span>
elements CSS will do the magic and our custom radio button image will be displayed in place of the default radio buttons. Also, don't forget to include the jQuery in your document before this script. We've called the customRadio()
function when DOM is fully loaded.
Example
Try this code »<script>
function customRadio(radioName){
var radioButton = $('input[name="'+ radioName +'"]');
$(radioButton).each(function(){
$(this).wrap( "<span class='custom-radio'></span>" );
if($(this).is(':checked')){
$(this).parent().addClass("selected");
}
});
$(radioButton).click(function(){
if($(this).is(':checked')){
$(this).parent().addClass("selected");
}
$(radioButton).not(this).each(function(){
$(this).parent().removeClass("selected");
});
});
}
// Calling customRadio function
$(document).ready(function (){
customRadio("browser");
});
</script>
Tip: If JavaScript is disabled in the browser, normal radio buttons will appear instead of customized radio buttons. So it will not break your code in any way.
Related FAQ
Here are some more FAQ related to this topic: