How to create custom checkboxes in HTML using CSS and jQuery
Topic: JavaScript / jQueryPrev|Next
Answer: Use the CSS :checked
Pseudo-class with jQuery
If you try to customize the checkboxes directly using the CSS properties like background
or border
it will not produce the desired result, because most of the form elements are native part of the
browsers and does not accepts most of the visual styling. So we have to find another way to style checkboxes.
In this tutorial we will create custom checkboxes that appears consistent across the browsers with the help of CSS and little bit of jQuery. There are many jQuery plug-ins available to customize checkbox elements but they are too complex and not so easy to integrate.
This solution will give you the full control over the style and placement of the checkboxes and very easy to implement in any project. Also, it is highly compatible across the browsers and work seamlessly even on the older version of the Internet Explorer such as IE7.
The HTML Code
Create the checkboxes through setting the type
attribute
to checkbox
on <input>
element and place in your HTML code. Now add the name
attribute to the <input>
elements with a
proper value, because it is required for this solution to work. The name
attribute is also
required if you want to get the values from these checkboxes using any server side script.
Example
Try this code »<form action="action.php" method="post">
<h3>Choose Your Favorite Sports</h3>
<label><input type="checkbox" name="sport[]" checked="checked" value="football"> Football</label>
<label><input type="checkbox" name="sport[]" value="cricket"> Cricket</label>
<label><input type="checkbox" name="sport[]" value="baseball"> Baseball</label>
<label><input type="checkbox" name="sport[]" value="tennis"> Tennis</label>
<label><input type="checkbox" name="sport[]" value="basketball"> Basketball</label>
</form>
Custom Checkbox Example Images
To keep this example simple and easy we have used different images to specify the different states of a checkbox like normal, hover, selected etc. But you should combine all these images in an image sprite form before implementing this solution in a real project for better performance and minimizing the HTTP request. However if you download the source code it will contain both the simple and sprite version of this custom checkbox example. Let's take a look at the following images.
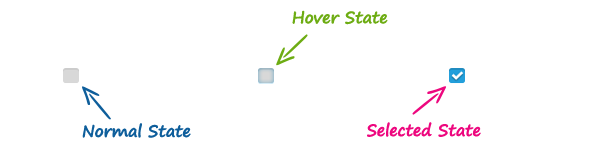
The CSS Code
What we are trying to achieve with the CSS is hiding the default checkboxes using the CSS opacity
property and display the custom checkboxes in place of them.
Now place the following style rules inside the head section of your HTML document.
Example
Try this code »<style>
.custom-checkbox{
width: 16px;
height: 16px;
display: inline-block;
position: relative;
z-index: 1;
top: 3px;
background: url("checkbox.png") no-repeat;
}
.custom-checkbox:hover{
background: url("checkbox-hover.png") no-repeat;
}
.custom-checkbox.selected{
background: url("checkbox-selected.png") no-repeat;
}
.custom-checkbox input[type="checkbox"]{
margin: 0;
position: absolute;
z-index: 2;
cursor: pointer;
outline: none;
opacity: 0;
}
</style>
You might be thinking we haven't used these classes anywhere in the HTML code; actually these classes will be added to the DOM by the jQuery at run time.
The jQuery Code
When you call the customCheckbox()
function it will wrap the <input>
element with a <span>
element and apply the .custom-checkbox
class on it. Once the .custom-checkbox
class is applied to the <span>
element CSS will hide the default checkbox and show the image of custom checkbox in place of them. Don't forget to include the jQuery file in your document before this script. We have called the customCheckbox()
function when DOM is fully loaded.
Example
Try this code »<script>
function customCheckbox(checkboxName){
var checkBox = $('input[name="'+ checkboxName +'"]');
$(checkBox).each(function(){
$(this).wrap( "<span class='custom-checkbox'></span>" );
if($(this).is(':checked')){
$(this).parent().addClass("selected");
}
});
$(checkBox).click(function(){
$(this).parent().toggleClass("selected");
});
}
$(document).ready(function (){
customCheckbox("sport[]");
});
</script>
Tip: If JavaScript is disabled in the browser, normal checkboxes will appear instead of customized checkboxes. So it will not break your code in any way.
Related FAQ
Here are some more FAQ related to this topic: